Kodeclik Blog
Python’s numpy ones() function
In your journey with Python numpy you will find a need to create arrays filled in 1s. One way to do it is to create an array of your desired dimensions and write a loop to fill it with 1s. Fear not, numpy has a handy function for it that does it for you in one line!
The numpy.ones() function takes several possible inputs: the desired dimensions, desired format (integer or float), the desired storage format (e.g., row-major or column-major ordering), and returns the array. Let us check out the various options!
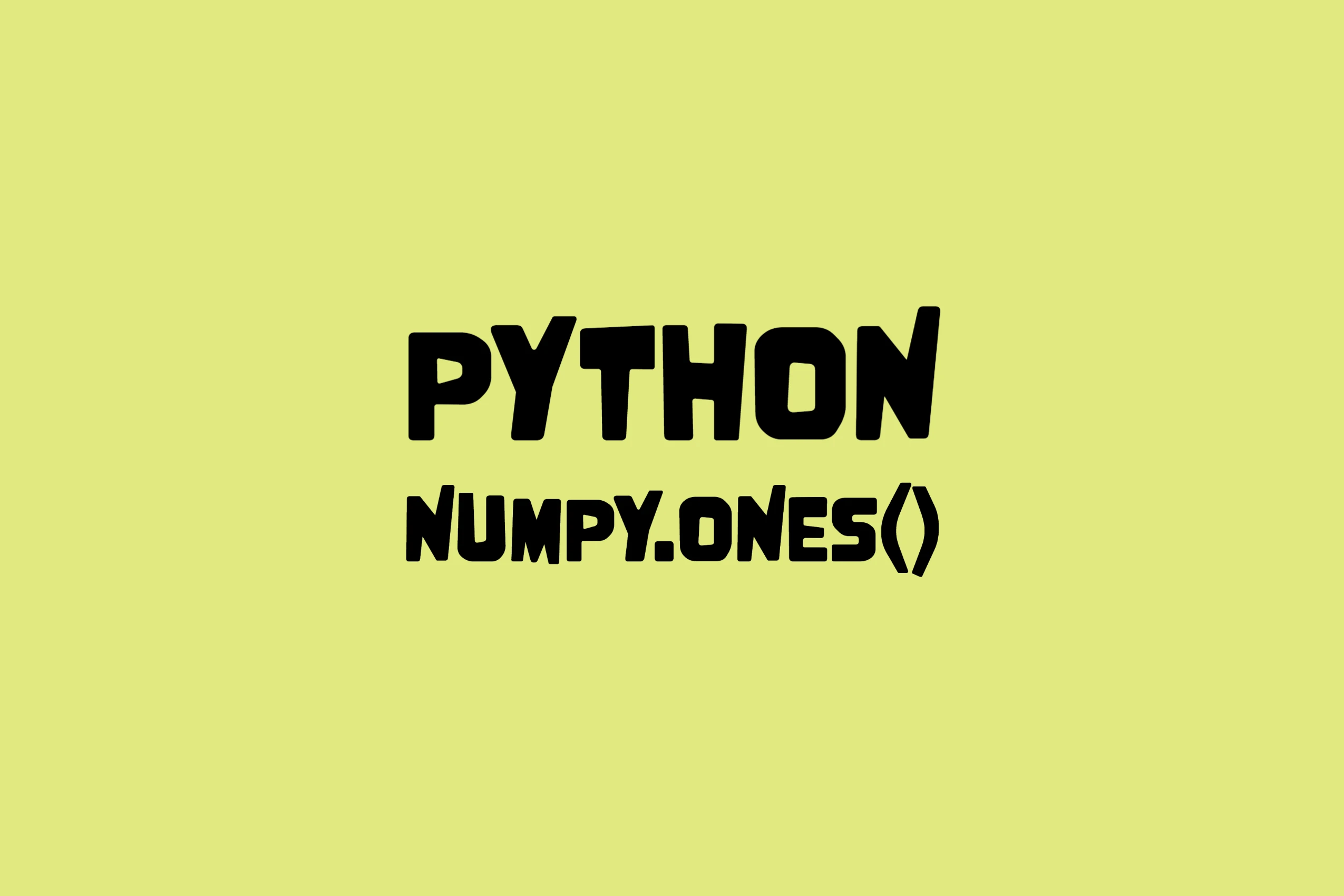
numpy.ones() to create a vector of 1s
The easiest way to use numpy.ones is to generate a vector (i.e., a single dimensional array) of 1s. Here is how that works:
import numpy as np
print(np.ones(5))
The output is:
[1. 1. 1. 1. 1.]
As you can see the one argument we gave to np.ones() is 5 and it has created a 5 dimensional vector of 1s, all floating point numbers.
numpy.ones() to create a two-dimensional array of 1s
We can similarly give two dimensions and create a two dimensional array of 1s:
import numpy as np
print(np.ones([5,4]))
The output is a 5 row x 4 column array of 1s:
[[1. 1. 1. 1.]
[1. 1. 1. 1.]
[1. 1. 1. 1.]
[1. 1. 1. 1.]
[1. 1. 1. 1.]]
numpy.ones() to create a three-dimensional array of 1s
What happens if we give three dimensions as input? Let us try it out!
import numpy as np
print(np.ones([5,4,2]))
The output is (note how long winding it is - because it is not possible to print a 3D array on a 2D screen) broken into 5 (first dimension) array slices of 4x2 each:
[[[1. 1.]
[1. 1.]
[1. 1.]
[1. 1.]]
[[1. 1.]
[1. 1.]
[1. 1.]
[1. 1.]]
[[1. 1.]
[1. 1.]
[1. 1.]
[1. 1.]]
[[1. 1.]
[1. 1.]
[1. 1.]
[1. 1.]]
[[1. 1.]
[1. 1.]
[1. 1.]
[1. 1.]]]
numpy.ones() with dtype parameter
In all the above examples, by default the entries are floats. You can specify you need integers by declaring an optional dtype parameter:
import numpy as np
print(np.ones([4,2],dtype=int))
The output is:
[[1 1]
[1 1]
[1 1]
[1 1]]
numpy.ones() with order parameter
Finally, numpy.ones() takes an order parameter that can be either ‘C’ or ‘F’. ‘C’ refers to a C (language)-style ordering, or row-major ordering. This means internally the arrays are stored in row-wise format. Similarly, ‘F’ refers to a Fortran (language)-style ordering, or column-major ordering. Internally, this means the arrays are stored in column-wise format. For our purposes, you will not notice any major differences when you print the arrays, but internally when numpy performs operations, some operations are better geared for column-major ordering vs. row-major ordering (depending on the operation), hence this choice. The default is column-major ordering wherein each column usually denotes a specific variable for which a lot of measurements have been taken (which are the rows).
Let us try varying this parameter and see what happens:
import numpy as np
print(np.ones([2,3],dtype=int,order='C'))
print(np.ones([2,3],dtype=int,order='F'))
The output is:
[[1 1 1]
[1 1 1]]
[[1 1 1]
[1 1 1]]
As you can see you cannot notice any difference (plus the sizes are too small). When you perform operations on large numpy arrays you will start noticing differences (depending on the operation).
If you liked this blogpost about numpy.ones, checkout our post on numpy.histogramdd() and about accessing pi in python numpy. Also learn how to compute cross-products in python numpy.
For more Python content, checkout the math.ceil() and math.floor() functions! Also learn about the math domain error in Python and how to fix it!
Interested in more operations to do with numpy vectors? Checkout outer products and how to create a square matrix from a vector!
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.