Kodeclik Blog
Formatting strings in Python
Formatting strings refers to pretty printing strings and Python provides multiple ways to format strings. Here are four ways to whet your appetite!
Method 1: The % operator
The most straightforward way to format strings is to use the % operator. It is easiest to illustrate how this works using an example. Consider the following code:
print('%s\'s sibling is %s')
It outputs:
%s's sibling is %s
You can think of “%s” as a placeholder, in this case for a string. This string therefore has two placeholders and to substitute values for these placeholders you must use the % operator and pass on two arguments, one for each of these placeholders. Here is an example:
print('%s\'s sibling is %s'%('Tommy','Jimmy'))
This outputs:
Tommy's sibling is Jimmy
Let us consider another example but this time instead of printing strings, we will print numbers using %d (for integers) and %f (for floating point numbers, or decimals).
num = 3
val = 1.5
print('%d apples at %f each cost %f.'%(num,val,num*val))
This outputs:
3 apples at 1.500000 each cost 4.500000.
You can control the resolution of the floating point numbers like so:
num = 3
val = 1.5
print('%d apples at %.2f each cost %.2f.'%(num,val,num*val))
This will output:
3 apples at 1.50 each cost 4.50.
Here is a Python program to print multiplication tables.
for x in [2,3]:
for y in range(12):
print('%d times %d is %d.'%(x,y+1,x*(y+1)))
This will output:
2 times 2 is 4.
2 times 3 is 6.
2 times 4 is 8.
2 times 5 is 10.
2 times 6 is 12.
2 times 7 is 14.
2 times 8 is 16.
2 times 9 is 18.
2 times 10 is 20.
2 times 11 is 22.
2 times 12 is 24.
3 times 1 is 3.
3 times 2 is 6.
3 times 3 is 9.
3 times 4 is 12.
3 times 5 is 15.
3 times 6 is 18.
3 times 7 is 21.
3 times 8 is 24.
3 times 9 is 27.
3 times 10 is 30.
3 times 11 is 33.
3 times 12 is 36.
Note how the numbers are not quite aligned because some of them are two digits and some are single digits. We can pretty print them, like so:
for x in [2,3]:
for y in range(12):
print('%2d times %2d is %2d.'%(x,y+1,x*(y+1)))
This will output:
2 times 1 is 2.
2 times 2 is 4.
2 times 3 is 6.
2 times 4 is 8.
2 times 5 is 10.
2 times 6 is 12.
2 times 7 is 14.
2 times 8 is 16.
2 times 9 is 18.
2 times 10 is 20.
2 times 11 is 22.
2 times 12 is 24.
3 times 1 is 3.
3 times 2 is 6.
3 times 3 is 9.
3 times 4 is 12.
3 times 5 is 15.
3 times 6 is 18.
3 times 7 is 21.
3 times 8 is 24.
3 times 9 is 27.
3 times 10 is 30.
3 times 11 is 33.
3 times 12 is 36.
Method 2: The format() method
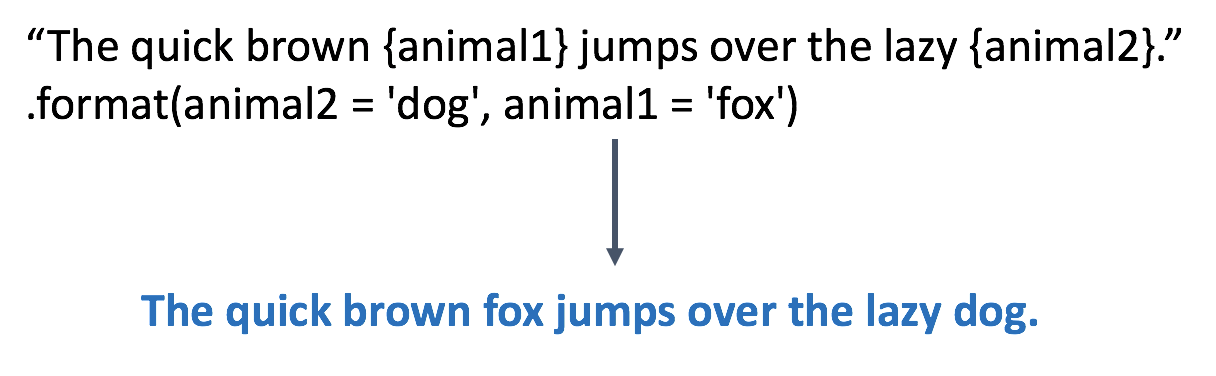
The format() method is the more modern way to format strings. It works as follows:
x = "The quick brown {} jumps over the lazy {}".format('fox','dog')
print(x)
Note that the curly braces denote placeholders and the arguments to the format() method denote the values that are substituted for these placeholders. Running this program yields:
The quick brown fox jumps over the lazy dog
Note that the number of arguments to format() must match the number of placeholders in the string. If we do:
x = "The quick brown {} jumps over the lazy {}".format('fox')
print(x)
We will get an error:
Traceback (most recent call last):
File "main.py", line 1, in <module>
x = "The quick brown {} jumps over the lazy {}".format('fox')
IndexError: Replacement index 1 out of range for positional args tuple
(Additional arguments will not cause an error.)
To make your code more readable, you can provide names for the placeholders.
x = "The quick brown {animal1} jumps over the lazy {animal2}"
.format(animal2 = 'dog', animal1 = 'fox')
print(x)
This will output:
The quick brown fox jumps over the lazy dog
Note that with this approach the order in which you pass arguments to format does not matter. The template has animal1 before animal2 but in the format argument we specify animal2 before animal1.
You can specify the same formatting instructions as before:
myfruits = 3
myrate = 1.5
myprice = myfruits * myrate
print("{num:d} apples at {rate:.2f} each cost {price:.2f}."
.format(num=myfruits, rate=myrate, price=myprice))
This will give rise to the output:
3 apples at 1.50 each cost 4.50.
Method 3: F-strings
F-strings are considered by many programmers to be the more natural way to do string formatting. Here’s how they work with the same example as above.
myfruits = 3
myrate = 1.5
myprice = myfruits * myrate
print(f"{myfruits} apples at {myrate} each cost {myprice}")
This will output:
3 apples at 1.5 each cost 4.5
Notice how the F-string expression literally substitutes the current known values of the variables in each placeholder location.
F-strings are also considered to be the faster way to format strings.
Method 4: Python string Template class
In this final approach, the notions of template and substitutes is made explicit using the Template class from the Python String module.
from string import Template
myfruits = 3
myrate = 1.5
myprice = myfruits * myrate
t = Template('$x apples at $y each cost $z.')
print (t.substitute({'x' : myfruits, 'y': myrate, 'z': myprice}))
This outputs:
3 apples at 1.5 each cost 4.5.
There you have it! You have learnt different ways to format strings - which one is your favorite?
Now that you know how to format strings, you can use the ideas you learnt here to send pretty formatted emails. Take a look at our Python send email post for more!
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.