Kodeclik Blog
How to pad a Python String
You will sometimes have the need to pad a Python string to fit a certain width. For instance, consider the following program:
name = "Kodeclik"
print(len(name))
The output is:
8
Let us suppose we wish to pad it so the total length is 12. There are three ways to pad the string. We can left justify it so the padding is on the right. Or, we can right justify it so the padding is on the left. Finally, we can center justify it so the padding is on both the left and right.
Method 1: Add padding to the right
In the first approach, we left justify the string using the ljust() method and specify the length of the new string as well as the padding character. Usually the padding character is a space but here we use another character just so that it is easy to observe the padding in action.
name = "Kodeclik"
print(name)
newname = name.ljust(12,"-")
print(newname)
In the above code, we are left justifying the string “name” with a length of 12, so four characters will be added as padding. These four characters will be represented as the character “-”. The output is thus:
Kodeclik
Kodeclik----
Method 2: Add padding to the left
In our second approach, we right justify the string using the rjust() method and again specify the length of the new string as well as the padding character. The corresponding code is:
name = "Kodeclik"
print(name)
newname = name.rjust(12,"-")
print(newname)
The output is:
Kodeclik
----Kodeclik
Once again we see that four dashes are added but this time to the left because we are using the rjust() method.
Method 3: Add padding on both left and right
To add padding to both left and right, we use the center() method on the string. The syntax for center() is exactly the same as for ljust() and rjust() above.
name = "Kodeclik"
print(name)
newname = name.center(12,"-")
print(newname)
The output is:
Kodeclik
--Kodeclik--
Thus, we have seen three methods to pad a Python string. Which one will you use and in what contexts?
Note that we have seen the most basic type of padding. We can get more creative as the situation demands. For instance here is a program to draw a tree using asterisk symbols:
for i in range(12):
for j in range(i):
print('**', end='')
print()
The output is:
**
****
******
********
**********
************
**************
****************
******************
********************
**********************
Note that we begin with two symbols and go on all the way to 22 (11*2=22) symbols at the bottom of the tree.
We can make this tree symmetric by using center padding. We can assume a total width of 32 for the purpose of this drawing:
for i in range(12):
row = ''
for j in range(i):
row = row + '**'
print(row.center(32, ' '))
Note that in the above program we first construct the string in variable “row” and then print this string by padding it using the center() method. The output is:
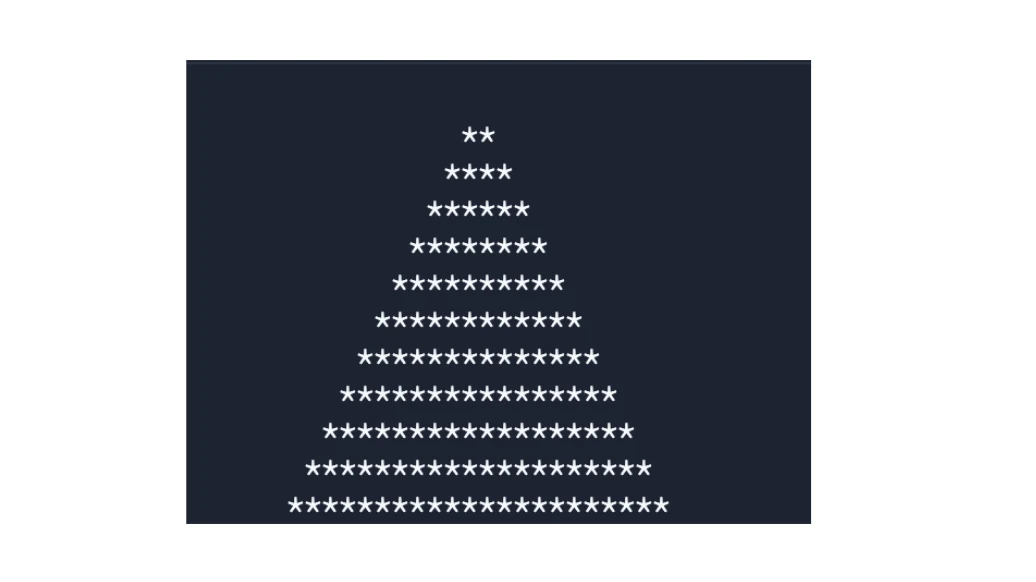
**
****
******
********
**********
************
**************
****************
******************
********************
**********************
giving us a nice centered tree.
To summarize, there are three ways to pad a Python string. You can use ljust() to left justify it so that the padding is on the right. You can use rjust() to right justify it so that the padding is on the left, or you can use center() to justify it in both directions so the padding is on both sides.
If you liked this blogpost, checkout blogpost on removing leading zeros from a Python string.
Interested in more things Python? Checkout our post on Python queues. Also see our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.